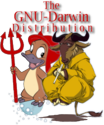
|
Main Page Namespace List Class Hierarchy Alphabetical List Compound List File List Compound Members File Members
gnBaseFeature Class ReferenceFeatures of DNA sequences can be accessed and manipulated using classes derived from gnBaseFeature.
More...
#include <gnBaseFeature.h>
Inheritance diagram for gnBaseFeature::
List of all members.
Public Methods |
| gnBaseFeature () |
| gnBaseFeature (string &name, uint32 id=0, gnFragmentSpec *spec=NULL, gnLocation::gnLocationType lt=gnLocation::LT_Nothing, boolean broken=false) |
| ~gnBaseFeature () |
| Destructor, frees memory. More...
|
virtual gnBaseFeature* | Clone () const=0 |
virtual string | GetName () const |
| Gets the feature name (e.g. More...
|
virtual void | SetName (const string &name) |
| Sets the feature name. More...
|
virtual uint32 | GetID () const |
| Gets this feature's ID. More...
|
virtual void | SetID (uint32 id) |
| Sets this feature's ID. More...
|
virtual gnFragmentSpec* | GetSpec () const |
| Gets the gnFragmentSpec to which this feature refers. More...
|
virtual void | SetSpec (gnFragmentSpec *spec) |
| Sets the gnFragmentSpec to which this feature refers. More...
|
virtual gnLocation::gnLocationType | GetLocationType () const |
| Gets this feature's location type. More...
|
virtual void | SetLocationType (gnLocation::gnLocationType lType) |
| Sets this feature's location type. More...
|
virtual uint32 | GetLocationListLength () const |
| Returns the number of locations this feature describes. More...
|
virtual boolean | AddLocation (const gnLocation &l, uint32 listI=0) |
| Adds a new location before the location at listI. More...
|
virtual gnLocation | GetLocation (uint32 listI) const |
| Gets the location at listI. More...
|
virtual boolean | RemoveLocation (uint32 listI) |
| Deletes the location at listI. More...
|
virtual boolean | SetLocation (const gnLocation &l, uint32 listI) |
| Sets the location at listI to a new location. More...
|
virtual boolean | MovePositive (const gnSeqI i) |
| Increases this feature's coordinates by a specific number of bases. More...
|
virtual boolean | MoveNegative (const gnSeqI i) |
| Decreases this feature's coordinates by a specific number of bases. More...
|
virtual boolean | CropStart (const gnSeqI i) |
| Crops the start locations of this feature by the specified amount. More...
|
virtual boolean | CropEnd (const gnSeqI i) |
| Crops the end locations of this feature by the specified amount. More...
|
virtual boolean | Crop (const gnLocation &l) |
| Crops the locations of this feature to fit within the given location. More...
|
virtual boolean | IsBroken () const |
| Returns true if the feature is broken. More...
|
virtual void | SetBroken (boolean broke) |
| Sets whether the feature is broken or not. More...
|
virtual boolean | Contains (gnSeqI i) const |
| Checks if the given coordinate is contained by this feature. More...
|
virtual boolean | Contains (const gnLocation &l) const |
| Checks if the given location is contained by this feature. More...
|
virtual boolean | Contains (gnBaseFeature *feature) const |
| Checks if the given feature is entirely contained by this feature. More...
|
virtual boolean | IsContainedBy (const gnLocation &l) const |
| Checks if this feature is entirely contained by the given location. More...
|
virtual boolean | Intersects (const gnLocation &l) const |
| Checks if the given location intersects this feature. More...
|
virtual boolean | Intersects (gnBaseFeature *feature) const |
| Checks if the given feature intersects this feature. More...
|
virtual uint32 | GetQualifierListLength () const |
| Returns the number of qualifiers in this feature. More...
|
virtual boolean | AddQualifier (gnBaseQualifier *qualifier) |
| Adds a new qualifier. More...
|
virtual boolean | HasQualifier (const string &name) const |
| Looks for a qualifier by name. More...
|
virtual uint32 | FirstIndexOfQualifier (const string &name, uint32 listI) const |
| Searches for a qualifier by name, starting at the index listI. More...
|
virtual uint32 | LastIndexOfQualifier (const string &name, uint32 listI) const |
| Searches for a qualifier by name, ending at the index listI. More...
|
virtual string | GetQualifierName (uint32 listI) const |
| Gets the name of the qualifier at the list index listI. More...
|
virtual string | GetQualifierValue (uint32 listI) const |
| Gets the value of the qualifier at the list index listI. More...
|
virtual gnBaseQualifier* | GetQualifier (uint32 listI) |
| Gets the qualifier at the list index listI. More...
|
virtual boolean | RemoveQualifier (uint32 listI) |
| Deletes the qualifier at the list index listI. More...
|
virtual boolean | SetQualifier (string &name, string &value, uint32 listI) |
| Set the name and value of the qualifier at the list index listI. More...
|
virtual boolean | SetQualifierName (string &name, uint32 listI) |
| Set the name of the qualifier at the list index listI. More...
|
virtual boolean | SetQualifierValue (string &value, uint32 listI) |
| Set the value of the qualifier at the list index listI. More...
|
Protected Attributes |
uint32 | m_id |
string | m_name |
boolean | m_broken |
gnLocation::gnLocationType | m_locationType |
vector< gnLocation > | m_locationList |
vector< gnBaseQualifier* > | m_qualifierList |
gnFragmentSpec* | m_spec |
Detailed Description
Features of DNA sequences can be accessed and manipulated using classes derived from gnBaseFeature.
gnBaseFeature outlines a basic interface and gives functionality its derived sequence classes, such as gnCDSFeature.
Definition at line 31 of file gnBaseFeature.h.
Constructor & Destructor Documentation
gnBaseFeature::gnBaseFeature (
|
)
|
|
gnBaseFeature::~gnBaseFeature (
|
)
|
|
Member Function Documentation
gnBaseFeature * gnBaseFeature::Clone (
|
) const [pure virtual]
|
|
boolean gnBaseFeature::Contains (
|
gnBaseFeature * feature ) const [virtual]
|
|
|
Checks if the given feature is entirely contained by this feature.
-
Parameters:
-
feature
|
The feature to check |
-
Returns:
-
True if "feature" is contained by this feature. False otherwise
Definition at line 145 of file gnBaseFeature.cpp. |
|
Checks if the given location is contained by this feature.
-
Parameters:
-
-
Returns:
-
True if "l" is contained by this feature. False otherwise
Definition at line 138 of file gnBaseFeature.cpp. |
|
Checks if the given coordinate is contained by this feature.
-
Parameters:
-
i
|
The coordinate to check |
-
Returns:
-
True if "i" is contained by this feature. False otherwise
Definition at line 130 of file gnBaseFeature.cpp. |
|
Crops the locations of this feature to fit within the given location.
-
Parameters:
-
l
|
The location to crop reduce to. |
-
Returns:
-
True if successful, false if the feature was broken by the change.
Definition at line 329 of file gnBaseFeature.h. |
|
Crops the end locations of this feature by the specified amount.
-
Parameters:
-
-
Returns:
-
True if successful, false if the feature was broken by the change.
Definition at line 59 of file gnBaseFeature.cpp.
Referenced by Crop(), and gnFragmentSpec::CropEnd().
|
|
Crops the start locations of this feature by the specified amount.
-
Parameters:
-
-
Returns:
-
True if successful, false if the feature was broken by the change.
Definition at line 68 of file gnBaseFeature.cpp.
Referenced by Crop(), and gnFragmentSpec::CropStart().
|
uint32 gnBaseFeature::FirstIndexOfQualifier (
|
const string & name,
|
|
uint32 listI ) const [virtual]
|
|
|
Searches for a qualifier by name, starting at the index listI.
-
Parameters:
-
name
|
The name of the qualifier to look for. |
listI
|
The index into the qualifier list to start the search at. |
-
Returns:
-
The index of the qualifier.
Definition at line 75 of file gnBaseFeature.cpp. |
uint32 gnBaseFeature::GetID (
|
) const [inline, virtual]
|
|
|
Gets this feature's ID.
-
Returns:
-
The feature's ID.
Definition at line 292 of file gnBaseFeature.h. |
uint32 gnBaseFeature::GetLocationListLength (
|
) const [inline, virtual]
|
|
|
Gets this feature's location type.
-
Returns:
-
The feature's location type.
-
See also:
-
gnLocationType
Definition at line 317 of file gnBaseFeature.h.
Referenced by gnGBKSource::Write().
|
string gnBaseFeature::GetName (
|
) const [inline, virtual]
|
|
|
Gets the qualifier at the list index listI.
-
Parameters:
-
listI
|
The index into the qualifier list. |
-
Returns:
-
The qualifier.
Definition at line 242 of file gnBaseFeature.cpp.
Referenced by gnGBKSource::Write().
|
uint32 gnBaseFeature::GetQualifierListLength (
|
) const [inline, virtual]
|
|
|
Returns the number of qualifiers in this feature.
-
Returns:
-
The number of qualifiers in this feature.
Definition at line 333 of file gnBaseFeature.h.
Referenced by gnGBKSource::Write().
|
string gnBaseFeature::GetQualifierName (
|
uint32 listI ) const [virtual]
|
|
|
Gets the name of the qualifier at the list index listI.
-
Parameters:
-
listI
|
The index into the qualifier list. |
-
Returns:
-
The name of the qualifier.
Definition at line 230 of file gnBaseFeature.cpp. |
string gnBaseFeature::GetQualifierValue (
|
uint32 listI ) const [virtual]
|
|
|
Gets the value of the qualifier at the list index listI.
-
Parameters:
-
listI
|
The index into the qualifier list. |
-
Returns:
-
The value of the qualifier.
Definition at line 236 of file gnBaseFeature.cpp.
Referenced by print_feature().
|
boolean gnBaseFeature::HasQualifier (
|
const string & name ) const [virtual]
|
|
|
Looks for a qualifier by name.
-
Parameters:
-
name
|
The name of the qualifier to look for. |
-
Returns:
-
True if a qualifier was found, false otherwise.
Definition at line 223 of file gnBaseFeature.cpp. |
boolean gnBaseFeature::Intersects (
|
gnBaseFeature * feature ) const [virtual]
|
|
|
Checks if the given feature intersects this feature.
-
Parameters:
-
feature
|
The location to check |
-
Returns:
-
True if "feature" intersects this feature. False otherwise
Definition at line 172 of file gnBaseFeature.cpp. |
boolean gnBaseFeature::IsBroken (
|
) const [inline, virtual]
|
|
uint32 gnBaseFeature::LastIndexOfQualifier (
|
const string & name,
|
|
uint32 listI ) const [virtual]
|
|
|
Searches for a qualifier by name, ending at the index listI.
-
Parameters:
-
name
|
The name of the qualifier to look for. |
listI
|
The index into the qualifier list to end the search at. |
-
Returns:
-
The index of the qualifier.
Definition at line 87 of file gnBaseFeature.cpp. |
|
Decreases this feature's coordinates by a specific number of bases.
-
Parameters:
-
i
|
The number of bases to decrease by. |
-
Returns:
-
True if successful, false if the feature was broken by the change.
Definition at line 50 of file gnBaseFeature.cpp. |
|
Increases this feature's coordinates by a specific number of bases.
-
Parameters:
-
i
|
The number of bases to increase by. |
-
Returns:
-
True if successful, false if the feature was broken by the change.
Definition at line 42 of file gnBaseFeature.cpp.
Referenced by gnGenomeSpec::GetFeature().
|
|
Deletes the location at listI.
-
Parameters:
-
listI
|
The index into the location list to delete. |
-
Returns:
-
True if successful, false otherwise.
Definition at line 199 of file gnBaseFeature.cpp. |
boolean gnBaseFeature::RemoveQualifier (
|
uint32 listI ) [virtual]
|
|
|
Deletes the qualifier at the list index listI.
-
Parameters:
-
listI
|
The index into the qualifier list. |
-
Returns:
-
True if successful, false otherwise.
Definition at line 97 of file gnBaseFeature.cpp. |
void gnBaseFeature::SetBroken (
|
boolean broke ) [inline, virtual]
|
|
|
Sets whether the feature is broken or not.
-
Parameters:
-
broke
|
True if the feature should be broken, false otherwise |
Definition at line 312 of file gnBaseFeature.h. |
void gnBaseFeature::SetID (
|
uint32 id ) [inline, virtual]
|
|
|
Sets the location at listI to a new location.
-
Parameters:
-
l
|
The new location |
listI
|
The index into the location list to set. |
-
Returns:
-
True if successful, false otherwise.
Definition at line 207 of file gnBaseFeature.cpp. |
void gnBaseFeature::SetName (
|
const string & name ) [inline, virtual]
|
|
boolean gnBaseFeature::SetQualifier (
|
string & name,
|
|
string & value,
|
|
uint32 listI ) [virtual]
|
|
|
Set the name and value of the qualifier at the list index listI.
-
Parameters:
-
name
|
The new name of the qualifier. |
value
|
The new value of the qualifier. |
listI
|
The index into the qualifier list. |
-
Returns:
-
True if successful, false otherwise.
Definition at line 104 of file gnBaseFeature.cpp. |
boolean gnBaseFeature::SetQualifierName (
|
string & name,
|
|
uint32 listI ) [virtual]
|
|
|
Set the name of the qualifier at the list index listI.
-
Parameters:
-
name
|
The new name of the qualifier. |
listI
|
The index into the qualifier list. |
-
Returns:
-
True if successful, false otherwise.
Definition at line 111 of file gnBaseFeature.cpp. |
boolean gnBaseFeature::SetQualifierValue (
|
string & value,
|
|
uint32 listI ) [virtual]
|
|
|
Set the value of the qualifier at the list index listI.
-
Parameters:
-
value
|
The new value of the qualifier. |
listI
|
The index into the qualifier list. |
-
Returns:
-
True if successful, false otherwise.
Definition at line 120 of file gnBaseFeature.cpp. |
void gnBaseFeature::SetSpec (
|
gnFragmentSpec * spec ) [inline, virtual]
|
|
Member Data Documentation
boolean gnBaseFeature::m_broken [protected]
|
|
uint32 gnBaseFeature::m_id [protected]
|
|
string gnBaseFeature::m_name [protected]
|
|
The documentation for this class was generated from the following files:
Generated at Fri Nov 30 15:36:53 2001 for libGenome by
1.2.8.1 written by Dimitri van Heesch,
© 1997-2001
|